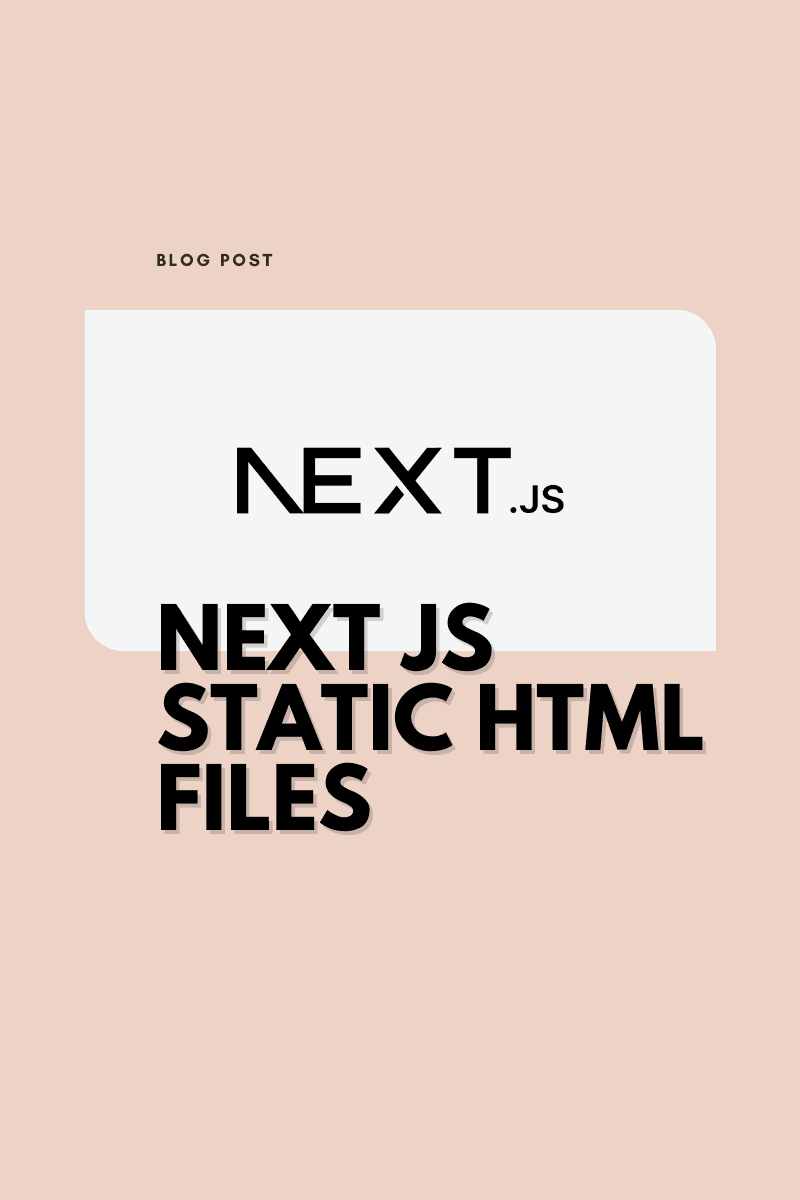
March 22nd 2023
Next JS
HTML
How to Add Static HTML Pages in Next.js
If for any reason you need to use static HTML pages in your Next JS application, this is the place for you. In my case, I created a form using ZOHO's webforms, and to use the form in my application, I needed to copy and paste the HTML, CSS, and JavaScript code they provided. To achieve this feature, it's a simple two-step process.
- Create the HTML file within the public folder
- Create a rewrite inside of the next.config file
Creating the HTML file within the public folder
This is a simple task. within the public folder of your next JS application, create an HTML file. The file can be nested inside another folder, as long as it is within the constraints of the public folder. This is helpful when adding proper structure to your code. so for the purpose of this example, we will add an html folder inside of the public folder, and create a file called index.html inside of that folder.
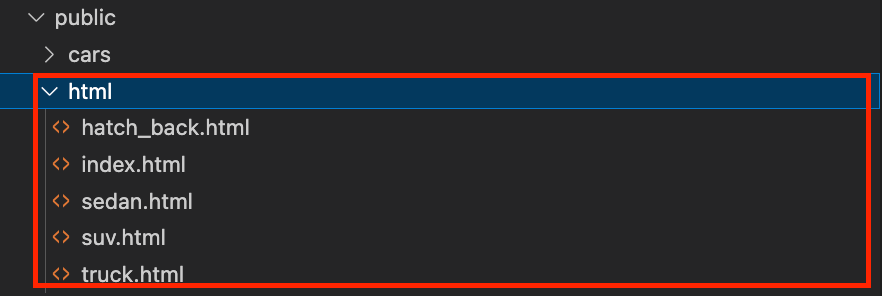
Create a rewrite inside of the next.config file
With step one completed, we can now open up the next.config file, and bring everything together. We need to add an async rewrites function, that returns an array. Inside this array, there are objects with source and destination key-value pairs. For the source key, you will need to enter the route at which you would like your HTML page to be rendered. For the destination key, you would need to enter the path to the html file.
// next.config.js
There are some things you must note when using these rewrites, the first being that the route you want to rebuild must not already be in the app's pages folder.
For instance, we cannot create a rewrite rule for a route if there is an existing file with the same route in the pages folder. Hence, in order for things to function, we would first need to rename or remove the existing file.
Also, you must use the Next.js path and not the relative path of the CSS, pictures, or other media assets if you wish to use native images or CSS files. For instance,
Actual format:
<img alt='some text' src='../img/asset.png' />
Next.js format:
<img alt='some text' src='img/asset.png' />
Since static files will be served from the Next.js application.
Conclusion
To use static HTML files in your Next JS application, you need to create the HTML file inside the public folder, then create a rewrite for Next JS to know where the file is, and which route to serve it.