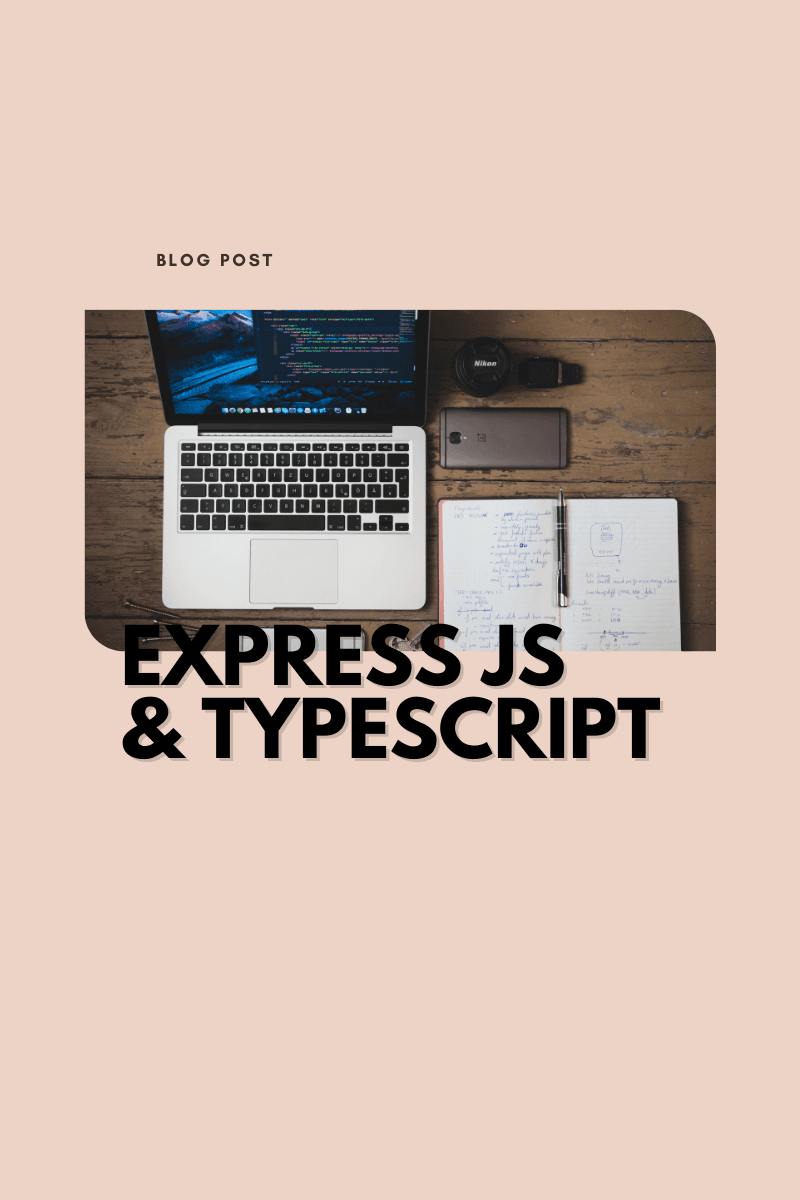
February 17th 2023
TypeScript
Express JS
Node JS
How to setup Express JS with TypeScript 2023 (Intermediate)
If you would like to build an API or the backend to your latest project using the Express JS framework and TypeScript, you've come to the right place. In this tutorial, I'll show you just how to get started in a few short steps.
- Create a project folder and initialise package.json
- Install project dependencies
- Initialise TypeScript
- Create an Express JS app
- Create scripts for production and development
- Quality of Life changes & folder structure suggestion
Create a project folder and initialise package.json
Each Node project's package.json file is its beating heart. It establishes the functional properties of a project that npm makes use of to set up scripts, install dependencies, and locate the entry point for our package. If you want to know more about this file, you will find the information here. We will be using it to install the dependencies and types needed for our project.
Open up your terminal, navigate to the desired directory, and run the following commands:
Install project dependencies
With the package.json initialised, we can now install all of the dependencies required for the application to work. Reopen the terminal, navigate to the express-typescript directory, and run the following commands:
You will now be able to use all these packages in your project.
Initialise Typescript
Run the following command:
If this step is not working for you, there will be information on how to solve it here. Most likely, it is because you do not have TypeScript installed on your machine.
Create an Express JS app
Open up the express-typescript folder in your code editor, and create a file titled index.ts. Import the express package inside of this file using es6 syntax, then create the app. Finally, you need to tell the app which port to listen on.
//index.ts
Create scripts for production and development
Open the package.json file you created and add the following scripts.
// package.json
You will also need to specify an output directory to match what was set in the scripts.
// tsconfig.json
You will now be able to run your Express application using TypeScript.
Quality of Life changes & folder structure suggestion
Instead of making an index.ts file, you can make a src folder where your entire app will live. In this folder, you will have other folders which contain routes, middleware, controllers, and other utility functions. You will also have a file in the root of the src directory labeled app.ts, the entry point of your application, and another file titled routes.ts which will house a function that creates all the routes present in your application.
Your folder structure should now resemble this:
-node_modules
-src
--controllers
--middleware
--routes
--utils
--app.ts
--routes.ts
-package.json
-tsconfig.json
Inside the routes folder, you can create the routes for your application. I will use a user route as an example. Create a file titled user.routes.ts, and create a simple route.
// src/routes/user.routes.ts
We will now move on to the routes.ts file located in the root of the src directory. We will create a routes function that accepts the express application as a parameter, and uses the application to create the routes.
//src/routes.ts
Inside the utils folder, you will create two files. One of which will be titled server.ts, which will be the file that creates your express server, and the other will be titled logger.ts, which will use the pino and dayjs libraries to make console log outputs look better, and easier to understand.
// src/utils/logger.ts
// src/utils/server.ts
With all of the setup done, we can now allow our application to begin listening on a specified port. This will be done inside the app file placed in the root directory of the src folder
// src/app.ts
These changes will make your code easier to scale, manage, and understand. However, there is one more important detail that we must not forget. Updating our scripts to work with the changes we made.
// package.json
Conclusion
We can easily create an express js application with TypeScript by following a few simple steps:
- Create a project folder and initialise package.json
- Install project dependencies and types
- Initialise TypeScript
- Create an Express JS app
- Create scripts for production and development